Mastering the Integration of Aarc Fund Deposit SDK
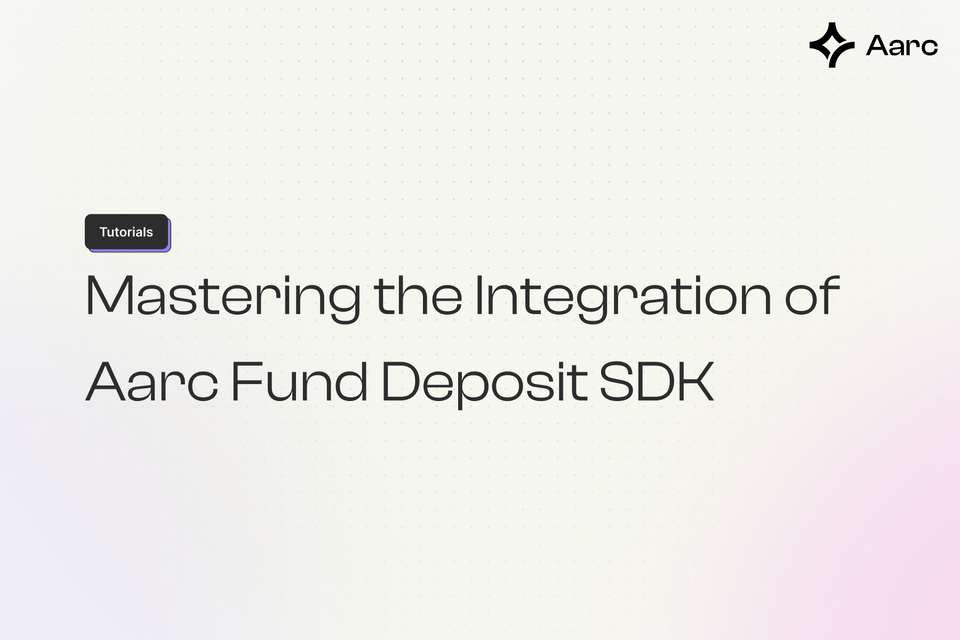
Web3, though designed for universal access, is not yet widely adopted due to its perceived complexity. However, it's not crypto itself that's complex; it's the surrounding infrastructure. This includes various L1 and L2 wallets, and exchanges, leading to scattered assets. Each entity has its own rules and interfaces, confusing newcomers.
To address this, recent efforts aim to simplify the crypto ecosystem and make it more integrated and interoperable. One such effort is the Aarc Fund Deposit Kit. It's designed to handle asset deposits from EOA wallets, exchanges and fiat(credit/debit cards) and streamline the process of onboarding funds into new smart wallets. It allows users to deposit their assets at a single destination, no matter what chain the assets are on.
The Aarc Fund Deposit Kit (FDK) is essential for improving the Developer Experience (DevX). Its simple SDK can be easily integrated into any web3 application, helping to unify asset aggregation and fund onboarding.
Aarc Fund Deposit Kit can be utilized in the following ways:
Let`s use the Aarc Fund Deposit Kit SDK to create a script for a cross-chain swap. This method is practical for managing digital assets across various blockchains. It improves the user experience and makes the deposit process more efficient, consolidating assets into one address without difficulty. Here are the steps and important components needed to use the performDeposit function of the Aarc Fund Deposit Kit SDK for these transactions.
Before You Begin
To prepare for your project using the Aarc Fund Deposit Kit SDK, ensure you have a few essentials ready.
Essentials:
- NPM: NPM is the backbone for managing project dependencies.
- JavaScript Fundamentals: As the Aarc Fund Deposit Kit SDK is likely JavaScript-based, a solid grasp of JavaScript's syntax and core concepts is crucial for effectively working with the SDK.
- Understanding Ethers.js: Familiarity with Ethers.js, a library for interacting with the Ethereum blockchain, will be incredibly beneficial since it's commonly used for blockchain communications, which are essential for working with the Aarc FDK SDK.
Getting Started
1.) Start by making a new directory, then initiate a new project with npm.
mkdir aarc-fdk-tutorial && cd aarc-fdk-tutorial && npm init -y
2.) Install the Aarc FDK SDK
npm i @aarc-xyz/core ethers@6.9
3.) Click Here to Obtain Your API Key for SDK Functionality
Setting up the Aarc Fund Deposit Kit SDK
- Create a new file
index.js
- Import the required modules
const ethers = require("ethers");
const {AarcCore} = require ("@aarc-xyz/core");
- Initialize the AarcCore SDK
const aarcSDK = new AarcCore("YOUR_AARC_API_KEY");
Defining Objects and Variables
- Create a signer object
const provider = new ethers.JsonRpcProvider("https://rpc-mainnet.maticvigil.com/");
const signer = new ethers.Wallet("YOUR_PRIVATE_KEY", provider);
- Create constants
const SOURCE_CHAIN_TOKEN_ADDRESS = "0x2791Bca1f2de4661ED88A30C99A7a9449Aa84174"; // USDC on Polygon PoS
const DESTINATION_CHAIN_TOKEN_ADDRESS = "0x82aF49447D8a07e3bd95BD0d56f35241523fBab1"; // WETH on Arbitrum
const SOURCE_CHAIN_ID = 137; // Polygon PoS
const DESTINATION_CHAIN_ID = 42161; // Arbitrum
const SOURCE_CHAIN_TOKEN_AMOUNT = 2000000; // 2 USDC
const RECIPIENT_ADDRESS = "0x1Cb30cb181D7854F91c2410BD037E6F42130e860";
Creating the function
- Create an async function
main
that will perform the deposit
async function main() {
const depositResponse = await aarcSDK.performDeposit({
sourceChainId: SOURCE_CHAIN_ID,
destinationChainId: DESTINATION_CHAIN_ID,
sourceChainTokenAddress: SOURCE_CHAIN_TOKEN_ADDRESS,
destinationChainTokenAddress: DESTINATION_CHAIN_TOKEN_ADDRESS,
sourceChainTokenAmount: SOURCE_CHAIN_TOKEN_AMOUNT,
recipientAddress: RECIPIENT_ADDRESS,
signer: signer
});
console.log(depositResponse);}
Here, the performDeposit
function takes the following parameters:
sourceChainId
: The chain ID of the source chaindestinationChainId
: The chain ID of the destination chainsourceChainTokenAddress
: The token address on the source chaindestinationChainTokenAddress
: The token address on the destination chainsourceChainTokenAmount
: The amount of tokens on the source chain to be depositedrecipientAddress
: The address of the recipient on the destination chainsigner
: The signer object
The complete script looks like this:
const ethers = require("ethers");
const {AarcCore} = require ("@aarc-xyz/core");
const aarcSDK = new AarcCore(
"YOUR_AARC_API_KEY"
);
const provider = new ethers.JsonRpcProvider("https://rpc-mainnet.maticvigil.com/");
const signer = new ethers.Wallet("YOUR_PRIVATE_KEY", provider)
const SOURCE_CHAIN_TOKEN_ADDRESS = "0x2791Bca1f2de4661ED88A30C99A7a9449Aa84174"; // USDC on Polygon PoS
const DESTINATION_CHAIN_TOKEN_ADDRESS = "0x82aF49447D8a07e3bd95BD0d56f35241523fBab1"; // WETH on Arbitrum
const SOURCE_CHAIN_ID = 137; // Polygon PoS
const DESTINATION_CHAIN_ID = 42161; // Arbitrum
const SOURCE_CHAIN_TOKEN_AMOUNT = 2000000; // 2 USDC
const RECIPIENT_ADDRESS = "0x1Cb30cb181D7854F91c2410BD037E6F42130e860";
async function main() {
try {
let response = await aarcSDK.performDeposit({
senderSigner: signer,
fromChainId: SOURCE_CHAIN_ID,
fromTokenAddress: SOURCE_CHAIN_TOKEN_ADDRESS,
toChainId: DESTINATION_CHAIN_ID,
toTokenAddress: DESTINATION_CHAIN_TOKEN_ADDRESS,
fromAmount: SOURCE_CHAIN_TOKEN_AMOUNT,
userAddress: signer.address,
recipient: RECIPIENT_ADDRESS
});
console.log("Response: ", response);
} catch (error) {
console.log("Error: ", error);
}
}
main();
Executing the script
To execute the above script, run the following command:
node index.js
Upon executing this function, Aarc SDK will check the user funds on the Source Chain, fetch the best possible routes from different providers, select a route, and perform the deposit on the user's behalf. All the user needs to do is sign the transactions.
Understanding the response
The response of executing the performDeposit
function will look like this:
[
{
tokenInfo: [ [Object] ],
type: 'ERC20_APPROVAL',
taskId: '',
status: 'Tx Sent',
txHash: '0xda9cafe77b32e59517310694954ebaafdcf68640defcd9fd47f490618d4528bf'
},
{
tokenInfo: [ [Object] ],
type: 'DEPOSIT',
taskId: '',
status: 'Tx Sent',
txHash: '0x63ca8d9a02f4730e6d6c127d0ae1086bad3a0e853478643f4eeec4583ccc5306'
}
]
There are two types of transactions in the response above:
ERC20_APPROVAL
: This transaction approves the cross-chain bridge provider to spend the user's tokens on the source chain.DEPOSIT
: This transaction executes the calldata on the source chain to deposit tokens on the destination chain using the cross-chain bridge.
Still facing issues? We've created a concise video guide to help you seamlessly integrate the Fund Deposit SDK into your dApp.
Video Tutorial for SDK Integration
All good to go now!
Thank you for building with us! To delve deeper into the Aarc Fund Deposit Kit SDK
We're here for you! Feel free to reach out if you have any questions or want to discuss anything.