Deposit API Integration: Your Step-by-Step Guide
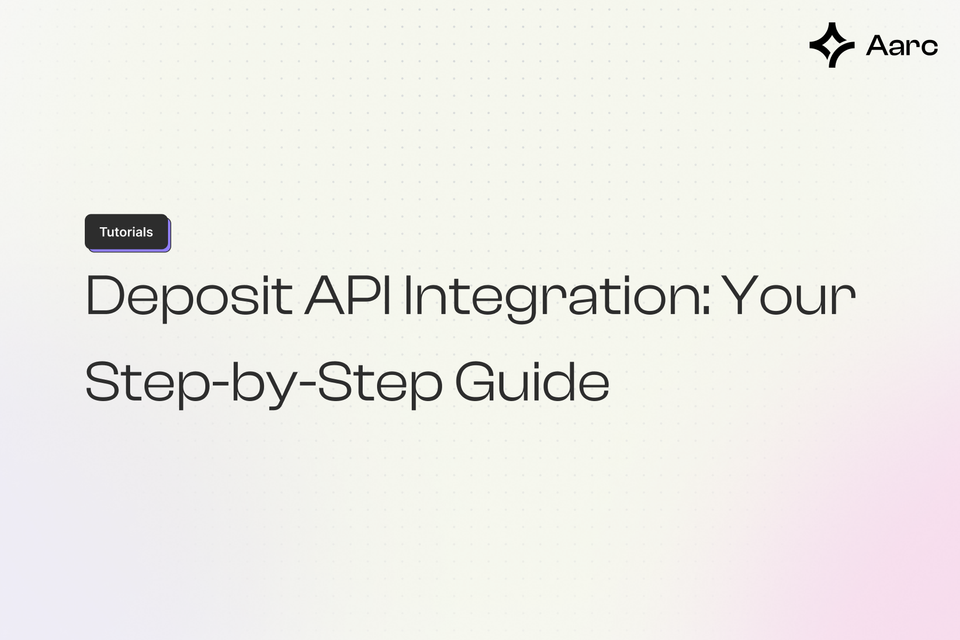
In the past year, numerous significant applications have built their dedicated app-specific chains or indicated plans to do so. Multiple fast-growing projects acknowledge the expected move towards appchains and rollups. They foresee a future where each major Web 3 application operates on its blockchain.
This narrative introduces complexities. The introduction of new rollups leads to a rise in the number of tokens, both new and existing, on these chains. This presents developers with the task of managing multiple tokens and chains, primarily identifying the chains and tokens used by their users.
Some challenging problems need to be addressed:
- For Application Developers
Integration Complexity: Supporting multiple chains increases the complexity of application architecture, requiring extensive knowledge about each chain's specifics, including consensus mechanisms, smart contract capabilities, and transaction models.
Bridging Complexity: Incorporating bridges for token transfers involves understanding the nuances of each bridge's operation, security mechanisms, and compatibility with target chains.
UI/UX Adjustments: Adapting the user interface to support multiple chains and bridges without compromising user experience is challenging. Developers must ensure the UI is intuitive and guides users through potentially complex processes. - For End User
Finding Reliable Bridges: Users must identify secure and efficient bridges for their assets, which requires research and trust in the bridge provider.
High Costs: Bridging tokens can incur significant fees, including gas costs and service fees, along with potential slippage in token value during the transfer process.
Transfer Delays: The time to complete a bridge transfer can vary widely, from a few minutes to several hours or even days, depending on network congestion and the bridge's mechanisms.
Aarc Deposit API
Aarc's Deposit API is a solution targeting the complexities and challenges faced by developers and users in the multi-chain ecosystem. Here's how it could address the issues highlighted earlier :
For Application Developers
- Comprehensive Transaction Data: By providing transaction data for the best or all possible routes for token bridging, Aarc helps developers optimize for cost, speed, and security. This data can be used to make informed decisions on which pathways to use for token transfers, potentially offering better rates and lower slippage to their users.
- Broad Chain and Token Support: With support for multiple chains and tokens, developers can expand their application's reach and utility without individually integrating each new chain or token. This opens up a wider audience and enhances the application's value proposition.
- No UI Changes Required: Since Aarc's solution does not necessitate changes to the application's UI, developers can maintain a seamless user experience. This is crucial for user retention and satisfaction, as it minimizes confusion and maintains ease of use even as new features and capabilities are added.
For Application Users
- Optimized Bridging Solutions: The application provides users access to optimized bridging routes, ensuring they get the best possible rates with minimal slippage and fees.
- Faster, More Reliable Transactions: With Aarc providing data on the best routes, users can expect faster and more reliable cross-chain transactions.
- Access to a Wider Range of Tokens and Chains: Applications can easily support multiple chains and tokens through Aarc's API, allowing users to access a broader ecosystem.
- Seamless User Experience: Integrating Aarc's solution without altering the UI allows users to enjoy a consistent and intuitive experience. This is particularly important for users who may not be technically inclined or familiar with the complexities of Web3.
Unlocking Cross-Chain Integration: How?
Prerequisites
Before proceeding with this tutorial, make sure you understand the following prerequisites:
Getting started
We're developing a swapping application with Next.js, incorporating :
- Utilizing Aarc Deposit API for cross-chain transactions
- Integrating RainbowKit for wallet connections
- To clone the repository and obtain the primary UI for the swapping application, execute the following command:
git clone https://github.com/aarc-xyz/fdk-api-tutorial-ui
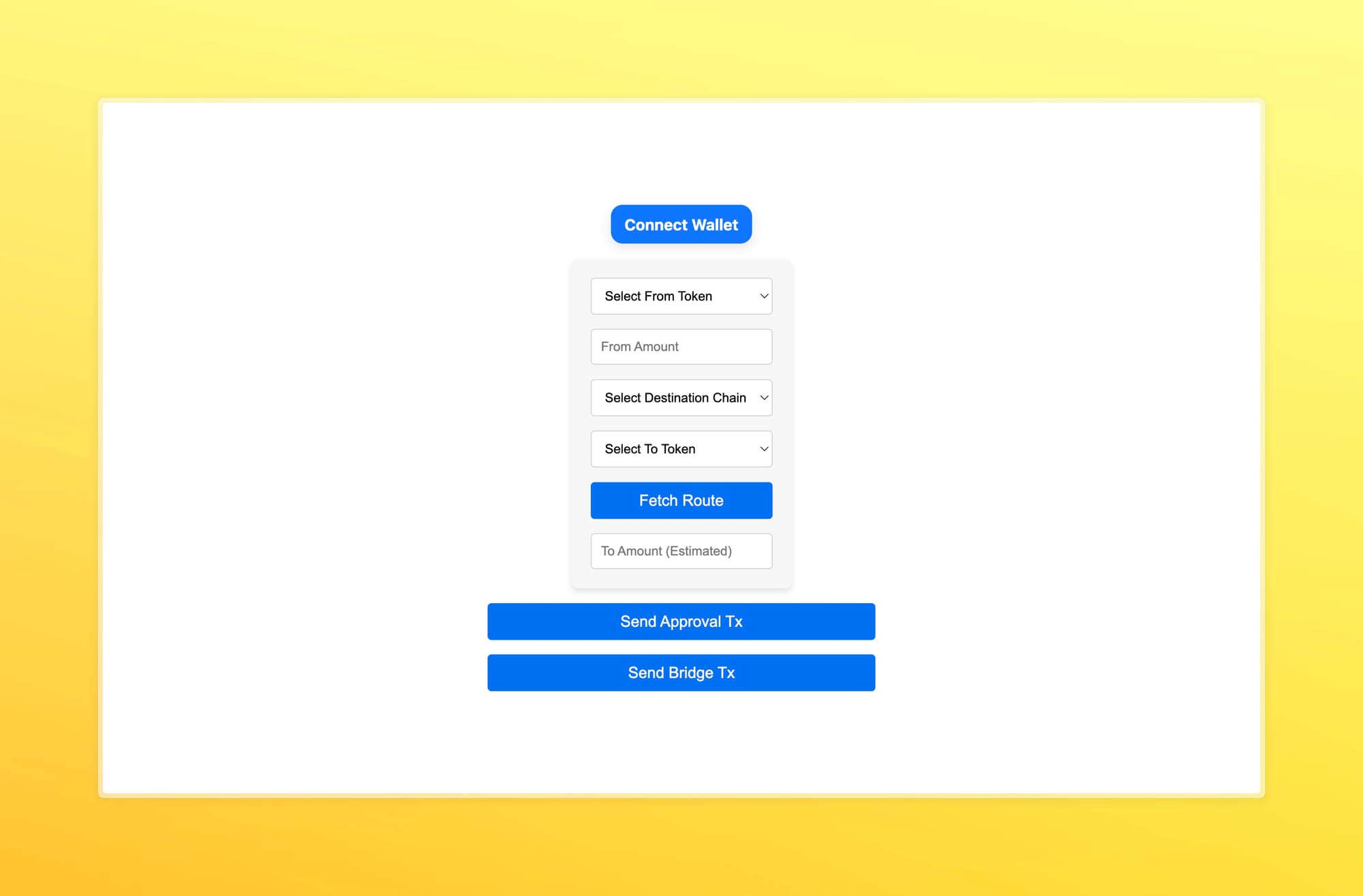
- Click Here to Obtain Your API Key for Cross-Chain Functionality
- Set up the environment variables
Create a .env.local
file in the root directory and add the following:
AARC_API_KEY=YOUR_AARC_API_KEY
Get the Wallet Connect API Key and paste it into the _app.js
file.
API Integration Essentials: Crafting Your Build
Having our UI ready, we'll build with the Aarc Depsit API.
- Initialize the directory.
Nextjs provides serverless functions to make API integration more accessible and more convenient. So, enter the following command in your terminal.
mkdir pages/api && cd pages/api
- Fetch the Deposit Calldata.
Create a new file namedindex.ts
in thepages/api
directory and add the following code:
import { NextApiRequest, NextApiResponse } from 'next';
import { ApprovalTx, ExecutionTxs, FinalResposne } from './types';
// base url for the cross-chain end point.
const BASE_URL = "https://bridge-swap.aarc.xyz";
// AARC API Key. Get it from here: https://dashboard.aarc.xyz/
const AARC_API_KEY = process.env.AARC_API_KEY ? process.env.AARC_API_KEY : "";
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
const {
recipient, routeType, fromChainId, fromTokenAddress, toChainId, toTokenAddress, fromAmount, userAddress
} = req.query;
const queryParams = new URLSearchParams({
recipient: recipient as string, // receiver address
routeType: routeType as string, // criteria to sort the routes
fromChainId: fromChainId as string, // source network chainId
fromTokenAddress: fromTokenAddress as string, // source token address on the source network
toChainId: toChainId as string, // destination network chainId
toTokenAddress: toTokenAddress as string, // destination token address on the destination network
fromAmount: fromAmount as string, // amount of token of `fromTokenAddress`
userAddress: userAddress as string // the owner of the token of `fromTokenAddress`
});
const endPoint = `${BASE_URL}/deposit-calldata?${queryParams}`; // final end point for the API
let response;
let responseInJson;
let finalResponse: FinalResposne = {
success: false,
data: {
approvalTxs: [],
executionTxs: []
}
};
try {
response = await fetch(endPoint, {
method: "GET",
headers: {
"x-api-key": AARC_API_KEY,
Accept: "application/json",
"Content-Type": "application/json",
},
});
} catch (error) {
console.error("Error fetching deposit calldata:", error);
}
if (response != undefined) {
try {
responseInJson = await response.json();
if (responseInJson.success) {
let approvalTxs = responseInJson.data.approvalTxs;
let executionTxs = responseInJson.data.executionTxs;
if (approvalTxs.length > 0) {
let approvalTxResponse: ApprovalTx;
approvalTxs.forEach((approvalTx: any) => {
approvalTxResponse = {
chainId: approvalTx.chainId,
txData: approvalTx.callData,
txTarget: approvalTx.approvalTokenAddress,
value: approvalTx.minimumApprovalAmount
};
finalResponse.data.approvalTxs.push(approvalTxResponse);
});
}
if (executionTxs.length > 0) {
let executionTxResponse: ExecutionTxs;
executionTxs.forEach((executionTx: any) => {
executionTxResponse = {
chainId: executionTx.chainId,
txData: executionTx.txData,
txTarget: executionTx.txTarget,
value: executionTx.value
};
finalResponse.data.executionTxs.push(executionTxResponse);
});
}
finalResponse.success = true;
}
else
console.error("Error fetching Deposit Calldata:", responseInJson);
} catch (error) {
console.error("Error parsing JSON response:", error);
}
}
res.status(200).json(finalResponse);
}
This will fetch the calldata required to execute the cross-chain swap. The calldata will include both the Approval Tx Calldata and the Execution Calldata.
The response to the above request:
{
"success": true,
"data": {
"approvalTxs": [
{
"chainId": "137",
"minimumApprovalAmount": "10000000",
"approvalTokenAddress": "0xc2132d05d31c914a87c6611c10748aeb04b58e8f",
"allowanceTarget": "0x3a23F943181408EAC424116Af7b7790c94Cb97a5",
"txType": "ERC20_APPROVAL",
"owner": "0x1cb30cb181d7854f91c2410bd037e6f42130e860",
"callData": "0x095ea7b30000000000000000000000003a23f943181408eac424116af7b7790c94cb97a50000000000000000000000000000000000000000000000000000000000989680",
"gasLimit": "59067"
}
],
"executionTxs": [
{
"chainId": "137",
"txData": "0x0000000346e0f5....00",
"txType": "DEPOSIT",
"txTarget": "0x3a23F943181408EAC424116Af7b7790c94Cb97a5",
"value": "0x00",
"gasLimit": "1000000"
}
]
}
}
That's it! Cheers, you've completed the API integration!
Thank you for building alongside us!
Aarc provides various solutions to improve the web3 experience for users and developers. For more information, we recommend checking out the Aarc Developer Docs.
We're here for you! Feel free to reach out if you have any questions or want to discuss anything.